VR Mesh Cutting
Company Academic Project |
Engine Unity |
||
Platform Windows PC, HTC Vive |
Skills C#, Graphics, Linear Algebra |
||
Role Gameplay Engineer |
Responsibilities Mesh Cutting, Sawing Behavior |
In-Game Videos
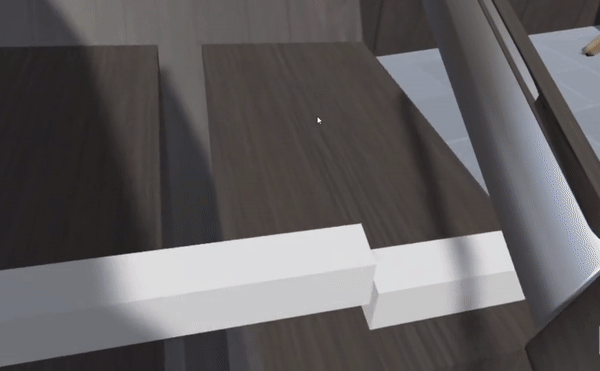
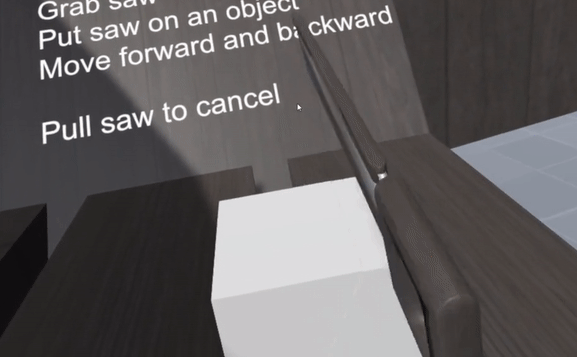
Experiment
Features
- Mesh Cutting with a Blade Width
- Concave Decomposition
- Optimization for Real-Time Performance
Convex Cutting
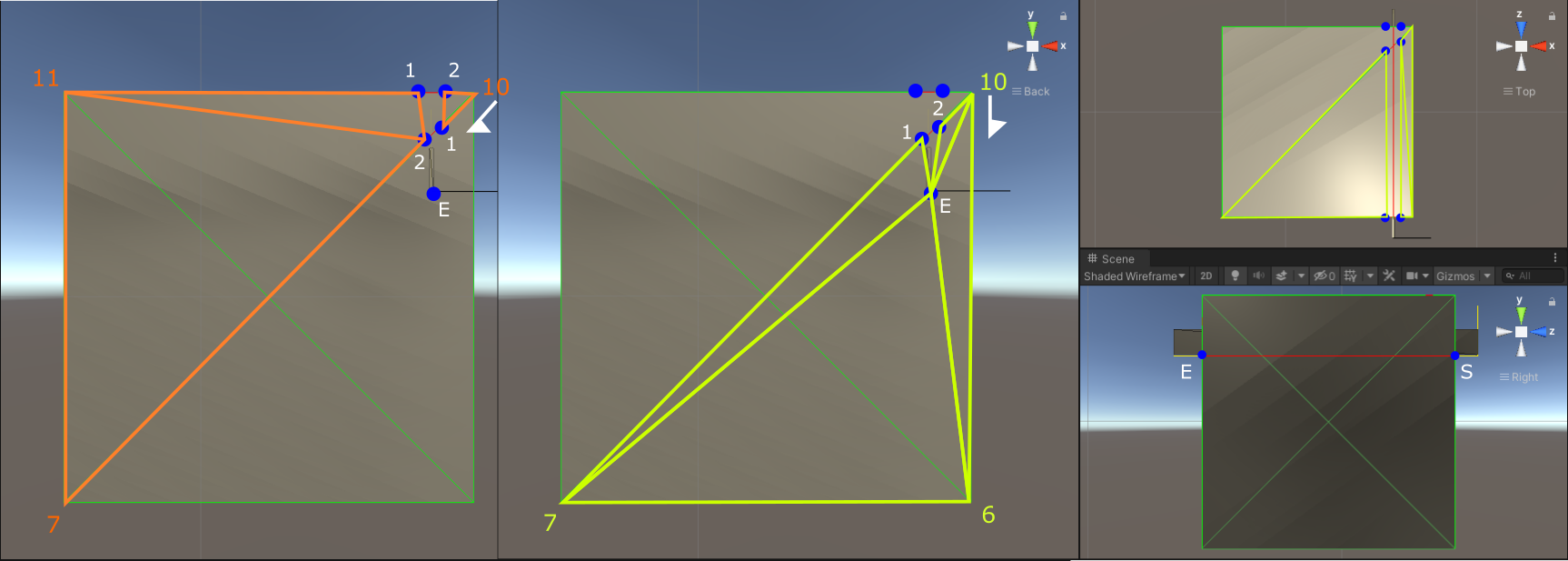
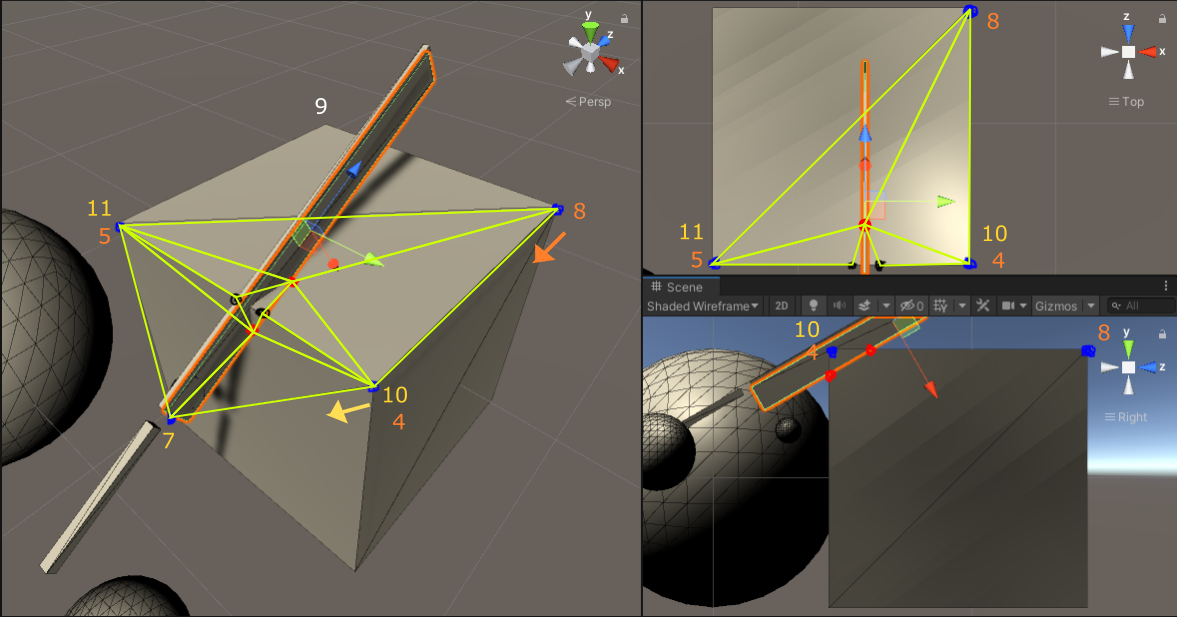
// The final data to rearrange vertex indices to split triangles
private struct StartEndPairPoints
{
CuttingDepthPoint start
CuttingDepthPoint end
List<CuttingStartPoints> cuttingPointsList
}
// Each depth point from a blade
private struct CuttingDepthPoint
{
// the start index in the triangle data of a mesh
int triangleIndex
// the intersected point between a ray and the object
float[3] point
float dist
// the start point of the sliced edge in clock-wised direction
float[3] pointStart
// the end point of the sliced edge in clock-wised direction
float[3] pointEnd
// the relative direction of the blade and the triangle order
bool isStartLeft
}
// Each sliced edge is refined as CuttingStartPoints
private struct CuttingStartPoints
{
int triangleStartIndex
// whether one edge is cut or not
int isolatedIndex
// the first vertex of a sliced edge in clock-wised order
int triangleIndex1
// the second one
int triangleIndex2
float[3] pointStart
float[3] pointEnd
bool isStartLeft
// whether the width of the blade is exactly on a vertex or not
bool isOnBlade
}
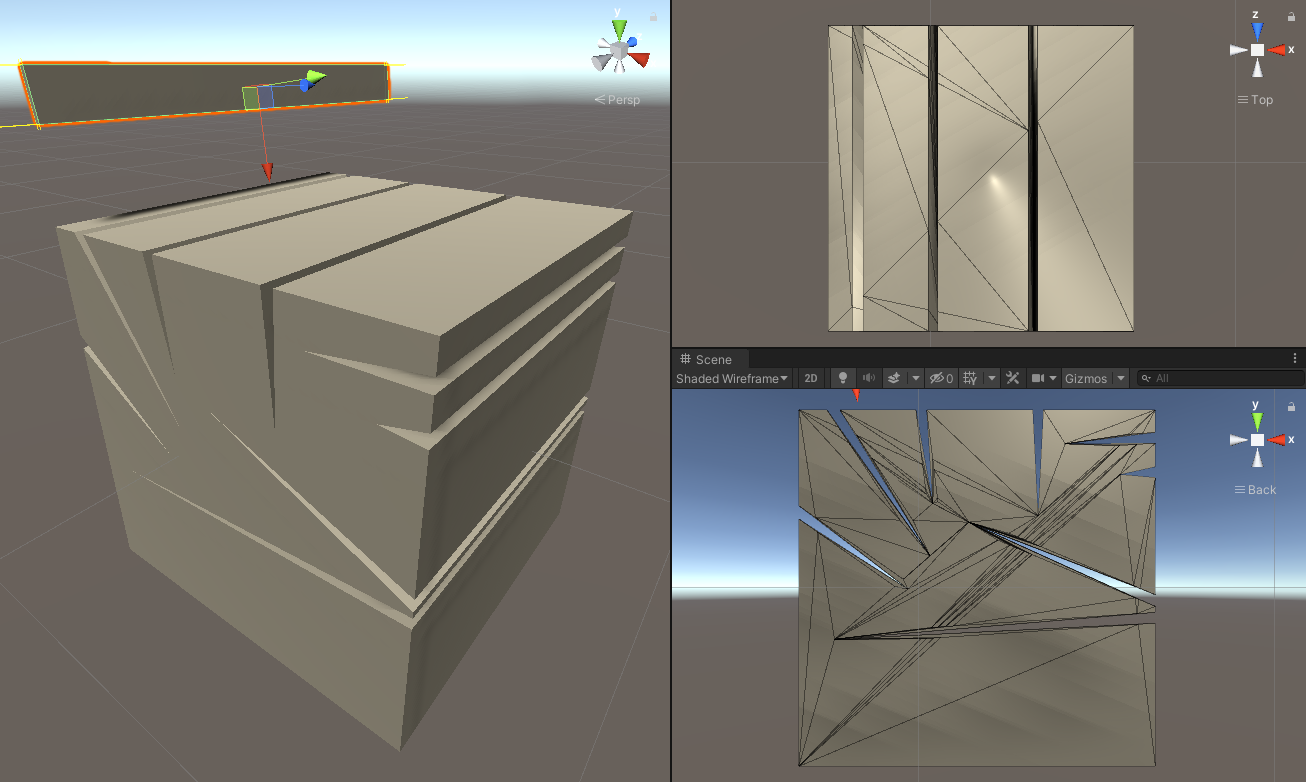
Concave Decomposition
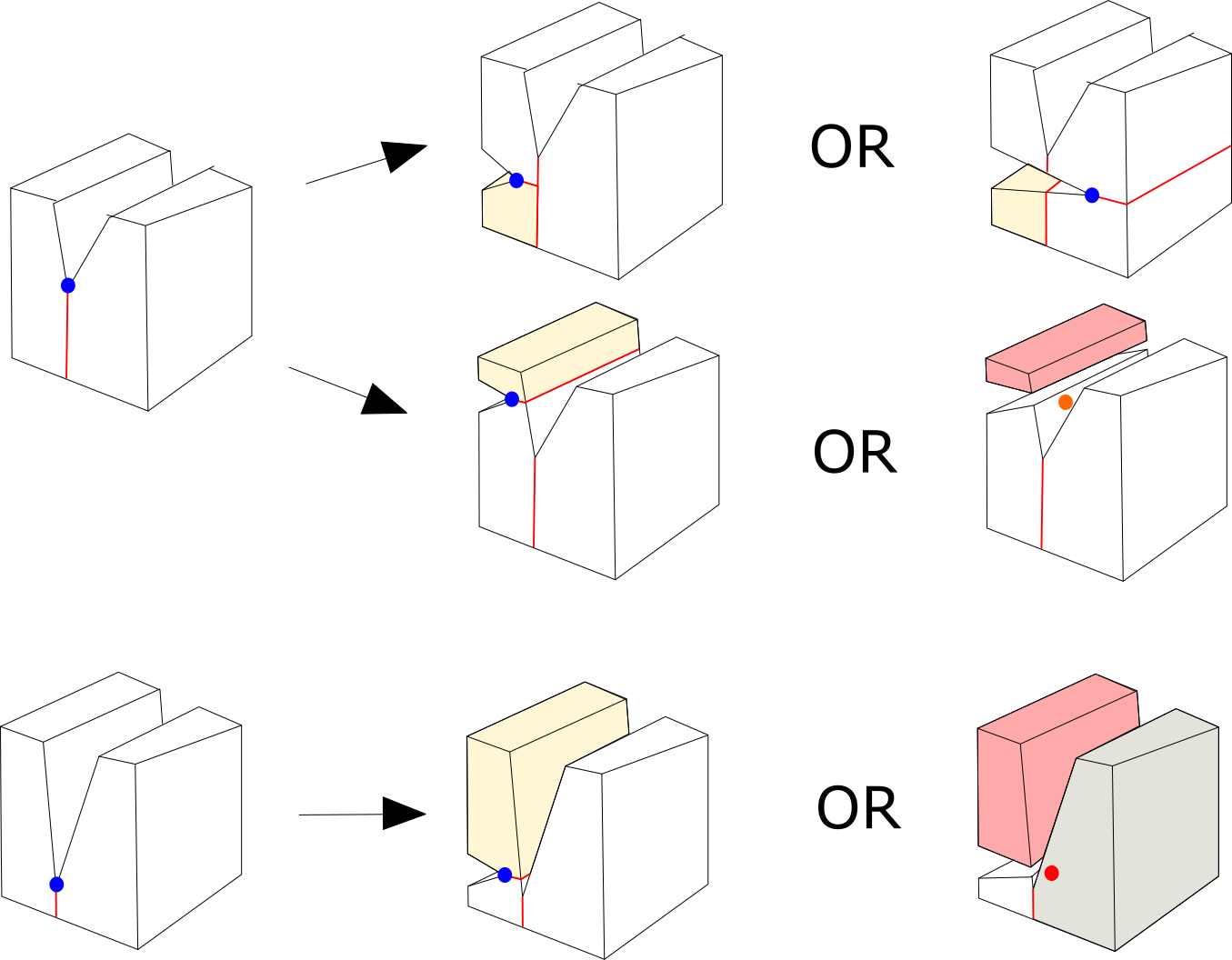
The above description is the main concept of the concave decomposition for this project. The blue and red dots are the depth point of each object. After an object is sliced into two convex objects, the two objects which are at the left and right side of the blade will test collision check with other objects only within the box.
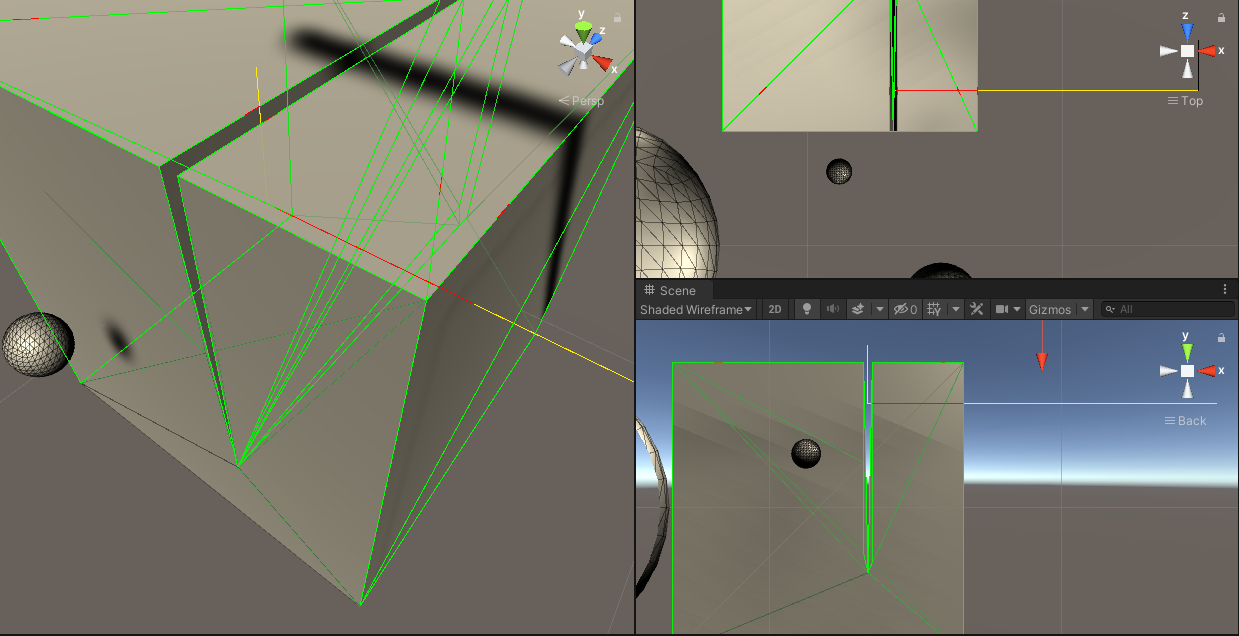
Optimization
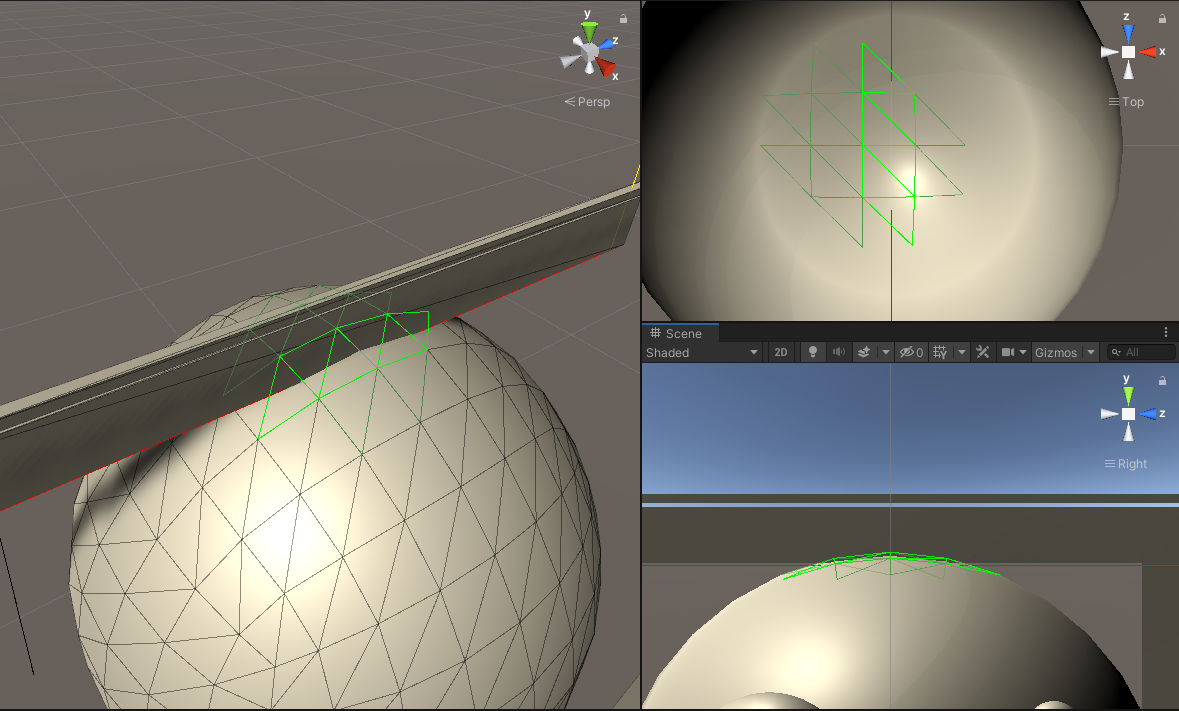
Reducing the calculation range is one of methods to optimize the performance. It partitions the space using a dot product between the perpendicular plane and each vertex so that only the upper trianges are conducted a complex calculation.